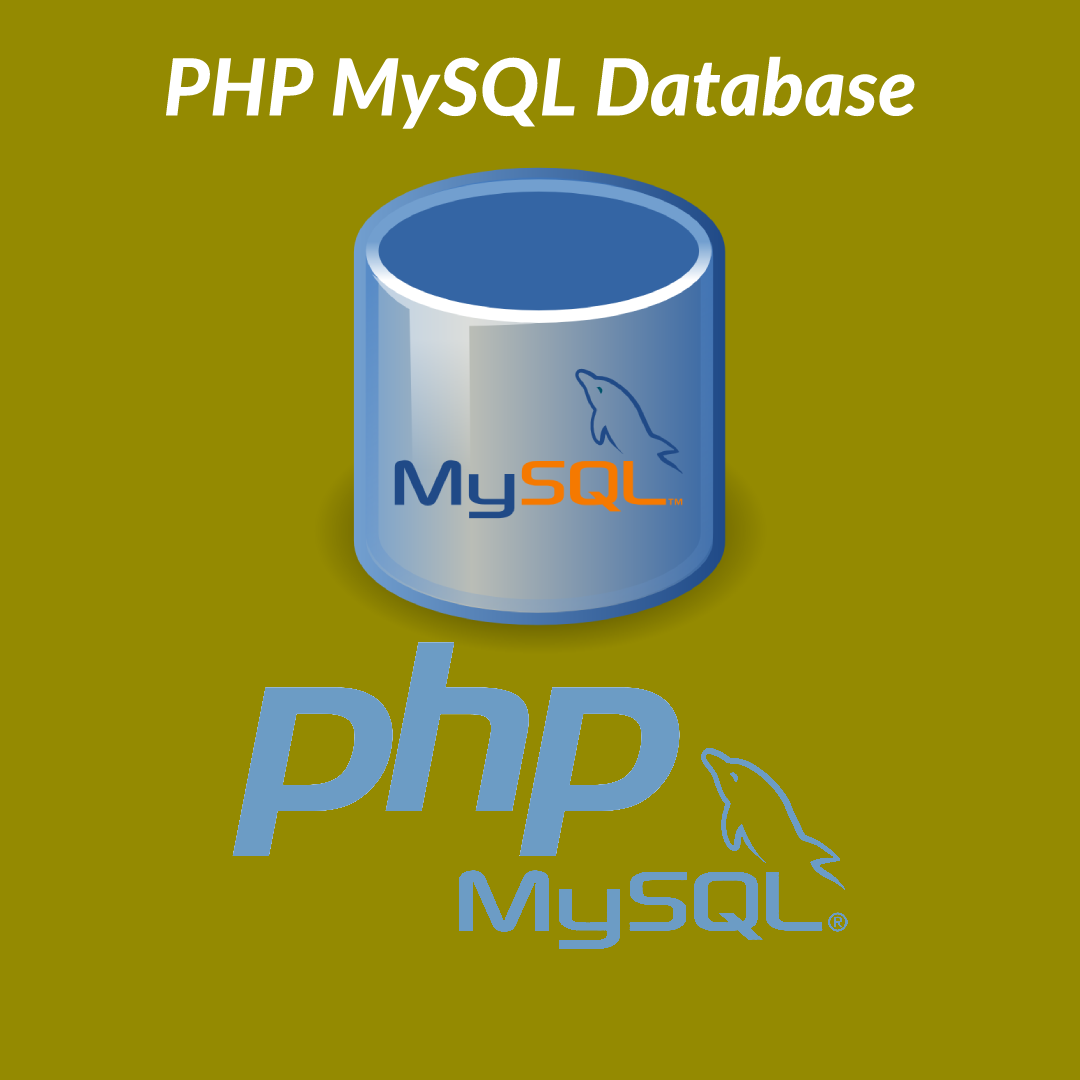
Connect PHP code with MySQL database and perform insert update and delete in wamp or xampp or lamp server
Today we are going to learn how to connect PHP code with MySQL database and perform insert update and delete in wamp or xampp or lamp server.
This would require a piece of MySQL information and online development surroundings using MySQL, Apache and PHP and a straightforward text editor.
The tutorial takes you through establishing a MySQL connection using PHP on an online page, connecting to a MySQL table and retrieving the results and displaying them back on the online page.
This tutorial uses the PHP MySQL commands:
mysqli_connect
mysqli_query
mysqli_fetch_array
mysqli_close
First Step: Connecting to a MySQL database
The code example is given below to connect with MySQL server using PHP,
Example:
<?php //Step1 $db = mysqli_connect('localhost','username','password','database_name') or die('Error connecting to MySQL server.'); ?>
The variable $db is created and assigned as the connection string, it will be used in future steps. If there is a failure then an error message will be displayed on the page. If it is successful you will see PHP connect to MySQL.
Second Step: Run a database query
The example of the query running process is given below,
Example:
<?php //Step1 $db = mysqli_connect('localhost','root','root','database_name') or die('Error connecting to MySQL server.'); //Step2 $query = "SELECT * FROM table_name"; mysqli_query($db, $query) or die('Error querying database.'); ?>
Again the returned page in the browser should be blank and error-free if you do receive the error – ‘Error querying database..’ check the table name is correct.
Put the info on the page
Here we tend to square measure taking the creating a $result variable that stores the question we tend to simply created on top of, currently we tend to simply ought to undergo all the rows of that question that we’d like mysqli_fetch_array that stores the rows in Associate in Nursing array, thus currently we tend to square measure storing the $result in mysqli_fetch_array and spending that into a variable known as $row.
The $row currently may be output in a very whereas loop, here the rows of information are echoed and displayed on the page to once there’s now not any rows of the information left, my example uses four fields within the table given name, last_name, email and town.
The code example is given below:
Example:
<?php //Step1 $db = mysqli_connect('localhost','root','root','database_name') or die('Error connecting to MySQL server.'); //Step2 $query = "SELECT * FROM table_name"; mysqli_query($db, $query) or die('Error querying database.'); $result = mysqli_query($db, $query); $row = mysqli_fetch_array($result); while ($row = mysqli_fetch_array($result)) { echo $row['first_name'] . ' ' . $row['last_name'] . ': ' . $row['email'] . ' ' . $row['city'] .'<br />'; } ?>
Here you should see all data as output from your table.
Closing the connection
Closing the association would require another set-out gap and shutting PHP tags once the closing hypertext markup language tag. it’s sensible to apply to shut the information association once the querying is finished.
The Example of the code is given below.
Example:
<?php //Step1 $db = mysqli_connect('localhost','root','root','database_name') or die('Error connecting to MySQL server.'); //Step2 $query = "SELECT * FROM table_name"; mysqli_query($db, $query) or die('Error querying database.'); //Step3 $result = mysqli_query($db, $query); $row = mysqli_fetch_array($result); while ($row = mysqli_fetch_array($result)) { echo $row['first_name'] . ' ' . $row['last_name'] . ': ' . $row['email'] . ' ' . $row['city'] .'<br />'; } //Step 4 mysqli_close($db); ?>
Database connections should be closed off. you are doing not got to keep the association variable $db once the initial association, however, is taken into account best apply.
I hope that the post Connect PHP code with MySQL database and perform insert update and delete in wamp or xampp or lamp server helped you, please provide your valuable comment below.
Thanks for Reading